In this blog we are discussing about the Exceptions and how to handle our custom exceptions in java.
First we need to know about the what is
Exception and what is
Error?
Exception is an abnormal condition which stops the execution of the program but an Error is sub class of
Throwable which indicates serious problems of the program.
See the below image for better understanding about the Hierarchy of Exception classes in java.
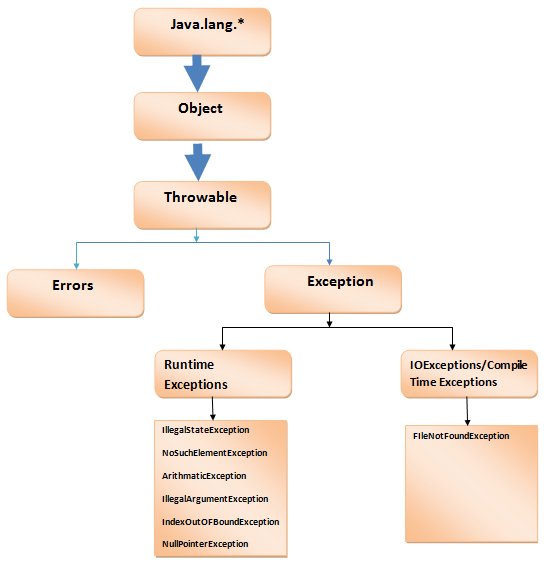 |
Exception class and its Hierarchy
|
Exception may occur at different
reasons such as like below.
1. User enters wrong input/ invalid
input.
2. Necessary file not found or cannot
found.
In java Exceptions may solve by
using try, catch and finally blocks.
Used key words in java for
handling Exceptions:
try, catch, finally, throw,
throws.
public class Exception
extends Throwable The class Exception and its subclasses are a form of Throwable that indicates conditions that a reasonable application
might want to catch.
The class Exception and any subclasses that are not also subclasses of RuntimeException are checked
exceptions. Checked exceptions need to be declared in a method or
constructor's throws clause if they can be thrown by the execution of the method
or constructor and propagate outside the method or constructor boundary.
Exceptions can be classified in
two ways.
1 1. Checked
Exceptions
2. Unchecked
Exceptions.
An unchecked exceptions, such as
an ArithmeticException
caused by attempting integer division
by 0, a NumberFormatException,
or a NullPointerException,
does not have to be handled with a try and catch block.
Checked Exceptions also known as Compile time Exceptions because these
are occurs at Compile time.
These exceptions are cannot
ignored, unless these are fixed programmer does not execute the program.
For example FileNotFoundException.
Syntax:
class A {
public static void main(String
args[]){
try{
statements;
}catch(Exception e){
statements;
}finally{
statements;
}
}
}
1. public Exception() - Constructs a new exception with null
as its detail message. The cause is not initialized, and may subsequently be initialized by a call to Throwable.initCause(java.lang.Throwable)
2. public Exception(String message)- Constructs a new exception with the specified detail
message. The cause is not initialized, and may subsequently be initialized by a
call to Throwable.initCause(java.lang.Throwable)
Parameters:
message - the detail message. The detail message is saved for later
retrieval by the Throwable.getMessage()
method
Constructs a new exception with the
specified detail message and cause. Note that the detail message associated
with cause is not automatically incorporated in this
exception's detail message.
Parameters:
cause
- the cause (which is saved for later retrieval by the Throwable.getCause()
method). (A null value is permitted, and indicates that the cause is
nonexistent or unknown.)
Since:
1.4
4. public Exception(Throwable cause)
Constructs a new exception with the
specified cause and a detail message of (cause==null ? null : cause.toString()) (which typically contains the class and detail message of cause).
This constructor is useful for exceptions that are little more than wrappers
for other throwables (for example, PrivilegedActionException).
Parameters:
cause
- the cause (which is saved for later retrieval by the Throwable.getCause()
method). (A null value is permitted, and indicates that the cause is
nonexistent or unknown.)
Since:
1.4
5. protected Exception(String message, Throwable cause,
boolean enableSuppression,boolean writableStackTrace)
Constructs a new exception with the
specified detail message, cause, suppression enabled or disabled, and writable
stack trace enabled or disabled.
Parameters:
message - the detail message.
cause
- the cause. (A null value is permitted, and indicates that the cause is
nonexistent or unknown.)
enableSuppression - whether or not suppression is enabled or disabled
writableStackTrace - whether or not the stack trace should be writable
Since:
1.7
Methods For Exception handling in java:
1. public
String getMessage() – it returns
string, the message is about the exception which occurs. This message is
initialized in the Throwable
constructor.
2.
public Throwable getCause() – it returns the problem of the exception which
is represented for Throwable object.
3.
public void printStackTrace() – it return the toString(), returns the result of error output stream.
4.
public StackTraceElement[] getStackTrace() - Provides
programmatic access to the stack trace information printed by printStackTrace(). Returns an array of stack trace elements, each
representing one stack frame. The zeroth element of the array (assuming the
array's length is non-zero) represents the top of the stack, which is the last
method invocation in the sequence. Typically, this is the point at which this
throwable was created and thrown. The last element of the array (assuming the
array's length is non-zero) represents the bottom of the stack, which is the
first method invocation in the sequence.
5.
public Throwable initCause(Throwable cause) -Initializes
the cause of this throwable to the specified value. (The cause is the
throwable that caused this throwable to get thrown.)
This
method can be called at most once. It is generally called from within the
constructor, or immediately after creating the throwable. If this throwable was
created with Throwable(Throwable)
or Throwable(String,Throwable),
this method cannot be called even once.
An
example of using this method on a legacy throwable type without other support
for setting the cause is: try {
lowLevelOp();
} catch (LowLevelException le) {
throw (HighLevelException)
new
HighLevelException().initCause(le);
//
Legacy constructor
}
6.
public String toString() - Returns a short description of this throwable. The result is
the concatenation of:
the name
of the class of this object
":
" (a colon and a space)
the result
of invoking this object's getLocalizedMessage()
method If getLocalizedMessage
returns null, then just the class name is returned.
Try with ARM (Automatic Resource Management) In java.